JavaScript Array Group
Grouping elements in an array is something you do all the time with your data..
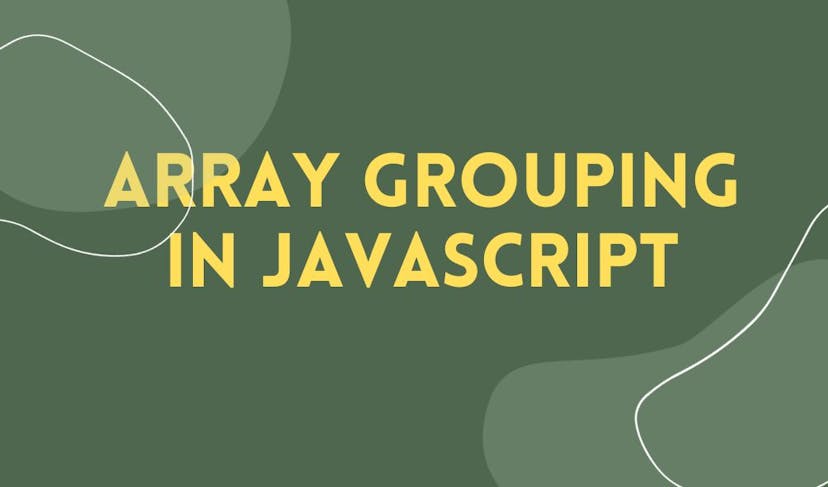
Introduction
Grouping elements in an array is something you do all the time with your data. Lodash's GroupBy has often done the job, but why use an ancillary library?
JavaScript will now have grouping methods, which will make your task easier. Object.groupBy and Map.groupBy are new methods that will make grouping easier and save us time or dependency.
The two groupBy methods are part of a TC39 proposal that is currently at stage 3. This means that there is a good chance it will become a standard and, as such, there are implementations appearing.
Let's work on it .
Object.groupBy()
You have a list of teams, where each team is an object having 2 properties: name and country.
const teams = [
{ name: "Liverpool", country: "England" },
{ name: "Manchester City", country: "England" },
{ name: "Paris SG", country: "France" },
{ name: "Real Madrid", country: "Spain" },
{ name: "Paris FC", country: "France" },
]
Now we want to group the clubs by country.
Object.groupBy returns an object where properties are teams names and values are arrays of team countries.
const groupByCountry = Object.groupBy(teams, (team) => {
return teams.country
})
/* Result is:
{
England: [
{ name: "Liverpool", country: "England" },
{ name: "Manchester City", country: "England" },
],
France: [
{ name: "Paris FC", country: "France" },
{ name: "Paris SG", country: "France" }
],
Spain: [
{ name: "Real Madrid", country: "Spain" }
]
}
*/
Grouping using teams.groupBy() is really easy to understand.
Object.groupBy(array, callback) accepts a callback function that's invoked with 3 arguments: the current array item, the index, and the array itself. The callback should return a string: the group name where you'd like to add the item.
Object.groupBy(items, callbackFn)
Map.groupBy()
Map.groupBy does almost the same thing as Object.groupBy except it returns a Map. This means that you can use all the usual Map functions. It also means that you can return any type of value from the callback function.
const data = [
{ name: "Sam", department: "IT" },
{ name: "Mehdi", department: "Marketing" },
{ name: "Oumar", department: "IT" },
]
const groupedByDepartment = Map.groupBy(data, (person) => person.department)
console.log(groupedByDepartment)
/* Result is:
Map({
"IT": [{ name: 'Sam', department: 'IT' }, { name: 'Oumar', department: 'IT' }],
"Marketing": [{ name: 'Mehdi', department: 'Marketing' }]
})
*/
While these methods are not entirely new (introduced in ES2020 - Jan 2020), they are still considered relatively new features and may not be familiar to all JavaScript developers. If want to use these functions right away, then use the polyfill provided by core-js library.